Introduction
API documentation for Profixio
This documentation aims to provide all the information you need to work with our API.
Pagination
While different methods require different data either as part of the URL, the query string or request body there are some parameters that are common to all API methods. These are passed as optional query parameters.
Mosts requests are paginated.
Query Parameters
page integer optional
The current page. Default is 1.
limit integer optional
Limit the number of records returned per page. Default is 15, the maximum is 500.
Paginated responses always contain meta and links keys with information about the paginator's state.
The data
field contains the actual data for the current page, while the meta field contains information about the pagination.
{
"data": [
{
"id": 1,
"name": "Eladio Schroeder Sr.",
"email": "[email protected]"
},
{
"id": 2,
"name": "Liliana Mayert",
"email": "[email protected]"
}
],
"links":{
"first": "http://example.com/users?page=1",
"last": "http://example.com/users?page=1",
"prev": null,
"next": null
},
"meta":{
"current_page": 1,
"from": 1,
"last_page": 1,
"path": "http://example.com/users",
"per_page": 15,
"to": 10,
"total": 10
}
}
Changelog
27.05.2025
- Added support for API keys scoped to a specific district (krets) for invoice endpoints. API keys can now be created for either an organisation (forbund) or a district (krets).
09.09.2024
- Added 'matchCategory' as filter to several endpoints (matches, tables, playerstatistics).
28.08.2024
- Added endpoints to get invoice info from the club license module in FX. To sync with external economy systems.
22.05.2024
- Added ability to update score in a match including set scores (Update match)
16.05.2024
- Added
seeding
number to TeamRegistrations using theseeding
parameter in the request body. - Added option (
include_referee_ids
) to include referee ids in league matches response. - Added
globalClubId
to Playerstatistics - Bugfix: Fixed playoffLevel in Match object
15.05.2024
- Added arenas endpoint to list all arenas in a tournament, including all fields
- Added endpoint to get all districts for an organisation
- Added
districtId
to tournament object - Added
teamId
to referees in tournament matches - Added
awayTeamPre
andhomeTeamPre
to Match object (to see playoff tree connections)
Authenticating requests
To authenticate requests, include a X-Api-Secret
header with the value "{YOUR_AUTH_KEY}"
.
All authenticated endpoints are marked with a requires authentication
badge in the documentation below.
Contact Profixio to get an API token.
Endpoints
Permissions
requires authentication
See what permissions your api key has
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/userinfo" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/userinfo';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/userinfo"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 119
{
"wsUser": {
"applicationName": "Odit et et modi.",
"contactEmail": "[email protected]",
"contactName": "Pearl Hauck Sr."
},
"permissions": {
"organisations": [
{
"organisationId": "NFF.NO.FB",
"viewTournament": true,
"listTournaments": false,
"updateAllTournaments": false,
"updateSpecificTournament": true,
"specificTournaments": [
{
"tournamentId": 12214,
"basicAccess": true,
"extendedAccess": true
}
]
}
]
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Sports
requires authentication
The sports registered in Profixio. An organisation may have one or several associated sports.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/sports?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/sports';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/sports"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 118
{
"data": [
{
"id": "BA",
"name": "bandy"
}
],
"links": {
"first": "https://www.profixio.com/api/sports?page=1",
"last": "https://www.profixio.com/api/sports?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/sports?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/sports",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
GET api/matches/{kamp}/setup
requires authentication
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/matches/architecto/setup" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/matches/architecto/setup';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/matches/architecto/setup"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (500):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 95
{
"message": "Server Error"
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Matches
Livescore
Match Event Types
requires authentication
Get a list of match event types in a organisation.
The types are used to categorize match events.
Some examples of match event types are:
- Goal (includes number of goals)
- Expulsion
- Fouls
- Start of match
- End of match
- Penalty competition
Period starts and stops
Events that starts/stops the match or periods includes 'gamestateStartStopInfo' in the event data.
Line up
Changes in team lineup can be submitted in three ways (not all are supported by all organisations):
- switches2Players: A single player is replaced by another player
- isActivatingOnePlayer: A single player is marked as active
- isTeamLineupMultiplePersons: Multiple players are replaced by multiple players. Includes "lineUp" in the event data.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SHF.SE.HB/matchEventTypes?sport=HB" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SHF.SE.HB/matchEventTypes';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'sport' => 'HB',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SHF.SE.HB/matchEventTypes"
);
const params = {
"sport": "HB",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 111
{
"data": [
{
"id": 186,
"sportId": "HB",
"parentId": null,
"name": "Matchstart",
"publicName": "Start första halvlek",
"isPublicEvent": true,
"isTeamEvent": false,
"isPersonEvent": false,
"numberOfPersonsInEvent": null,
"isGoalEvent": false,
"numberOfGoals": null,
"isTeamFoul": false,
"isPersonFoul": false,
"givesTimeout": false,
"removesTimeout": false,
"isPenaltyCompetition": false,
"allowsComment": false,
"penaltyDuration": {
"minutesLimit": null,
"minutesForPeriodsLessThanLimit": 0,
"minutesForPeriodsGreaterOrEqualToLimit": 0
},
"isExpulsion": false,
"isFullTimeExpulsion": null,
"isYellowCard": false,
"assignablePlayer": false,
"assignableCoach": false,
"assignableAssistantCoach": false,
"benchForAttacks": null,
"startsTimer": false,
"stopsTimer": false,
"allowsReport": false,
"gamestateStartStopType": 1,
"gamestateStartStopInfo": {
"startsMatch": true,
"stopsPeriod": false,
"startsPeriod": false,
"stopsMatch": false,
"startsExtraPeriod": false,
"stopsExtraPeriod": false
},
"pointForOtherTeam": false,
"switches2Players": false,
"isActivatingOnePlayer": false,
"isTeamLineupMultiplePersons": false,
"sign": null,
"addsStoppageTimeForPeriod": false
},
{
"id": 187,
"sportId": "HB",
"parentId": null,
"name": "Halvlek slut",
"publicName": "%%omgang%%:a halvlek är slut",
"isPublicEvent": true,
"isTeamEvent": false,
"isPersonEvent": false,
"numberOfPersonsInEvent": null,
"isGoalEvent": false,
"numberOfGoals": null,
"isTeamFoul": false,
"isPersonFoul": false,
"givesTimeout": false,
"removesTimeout": false,
"isPenaltyCompetition": false,
"allowsComment": false,
"penaltyDuration": {
"minutesLimit": null,
"minutesForPeriodsLessThanLimit": 0,
"minutesForPeriodsGreaterOrEqualToLimit": 0
},
"isExpulsion": false,
"isFullTimeExpulsion": null,
"isYellowCard": false,
"assignablePlayer": false,
"assignableCoach": false,
"assignableAssistantCoach": false,
"benchForAttacks": null,
"startsTimer": false,
"stopsTimer": false,
"allowsReport": false,
"gamestateStartStopType": 2,
"gamestateStartStopInfo": {
"startsMatch": false,
"stopsPeriod": true,
"startsPeriod": false,
"stopsMatch": false,
"startsExtraPeriod": false,
"stopsExtraPeriod": false
},
"pointForOtherTeam": false,
"switches2Players": false,
"isActivatingOnePlayer": false,
"isTeamLineupMultiplePersons": false,
"sign": null,
"addsStoppageTimeForPeriod": false
}
]
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Match lineup / staff and players
requires authentication
Get the lineup for a match. Available some minutes before match start. Varies from tournament to tournament.
See the match->lineupAvailableFrom field.
If called before x minutes, the server responds with a 403 Forbidden.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/matches/30843160/lineup" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/matches/30843160/lineup';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/matches/30843160/lineup"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
{
"data": {
"home": {
"staff": [
{
"id": 655735,
"addedManually": false,
"firstName": "John",
"lastName": "Doe",
"personId": 48668812,
"birthDate": '1980-01-02',
"role": {
"id": 2,
"name": "Coach"
}
}
],
"players": [
{
"id": 864135,
"number": "1",
"addedManually": false,
"firstName": "Kenny",
"lastName": "Maduro",
"position": null,
"personId": 332342,
"birthDate": "2002-05-11",
"isCaptain": false
},
{
"id": 8642232,
"number": "2",
"addedManually": true,
"firstName": "Cristiano",
"lastName": "Ronaldo",
"position":
{
"id": 4,
"name": "MV",
"goalkeeper": true
}
"personId": null,
"birthDate": null,
"isCaptain": false
}
]
},
"away": {
"staff": [],
"players": []
}
}
}
Example response (403, Lineup not available yet):
{
"message": "Lineup not available yet"
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Match Protocol Events
requires authentication
List of match events. Most recent events first.
The event types vary from sport to sport.
Events of type "gamestateStartStopType" are used to indicate the start and end of a game or period.
Some events are team specific, and some are player/staff specific. The teamId field indicates which team the event belongs to. The "person" field is only set for player/staff specific events.
Some events also have a second person, for example a player who is substituted, or a goal with an assist. The "person2" field is only set for events with a second person.
In some cases, goals does not have a person field. This means that the secretary has not yet entered the player who scored the goal.
All events include the current score.
Game time: Some matches have a match clock, some may not. The "totalGameTime", "timeInPeriod" and "overtimeInperiod" fields are only set for matches with a match clock.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/34301/matches/31553763/events" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/34301/matches/31553763/events';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/34301/matches/31553763/events"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 96
{
"data": [
{
"id": 1108827,
"eventTypeId": 189,
"description": "Matchen är slut",
"isTeamEvent": false,
"teamId": null,
"teamName": null,
"currentScore": {
"home": 13,
"away": 44
},
"isShootoutRound": false,
"shootoutScore": null,
"totalGameTime": "60:00",
"displayGameTime": "60:00",
"period": 2,
"timeInPeriod": "30.00",
"overtimeInperiod": "0.00",
"comment": null,
"goals": null,
"symbol": "",
"timeout": false,
"startsPenaltyCompetition": false,
"isExpulsion": false,
"penalty": null,
"switches2Players": false,
"isTeamLineupMultiplePersons": false,
"isActivatingOnePlayer": false,
"gamestateStartStopType": 4,
"isTeamFoul": false,
"isPersonFoul": false,
"hasReport": false,
"created_at": "2023-08-19T14:28:20.000000Z",
"sortOrder": 2300000,
"startedAt": "2023-08-19T14:28:20.000000Z",
"pausedAt": null,
"addSeconds": null,
"startsMatch": false,
"stopsPeriod": false,
"startsPeriod": false,
"stopsMatch": true,
"startsExtraPeriod": false,
"stopsExtraPeriod": false
}
]
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Seasons
Matches in a season
requires authentication
Get a list of matches in a season
See Tournament -> Matches for matches for a specific tournament.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/seasons/711/matches?updated=2022-10-24+08%3A00%3A00&fromDate=2022-10-01&toDate=2022-10-05+14%3A40%3A00&include_referee_ids=1&matchCategory=3247289&limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/seasons/711/matches';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'updated' => '2022-10-24 08:00:00',
'fromDate' => '2022-10-01',
'toDate' => '2022-10-05 14:40:00',
'include_referee_ids' => '1',
'matchCategory' => '3247289',
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/seasons/711/matches"
);
const params = {
"updated": "2022-10-24 08:00:00",
"fromDate": "2022-10-01",
"toDate": "2022-10-05 14:40:00",
"include_referee_ids": "1",
"matchCategory": "3247289",
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 106
{
"data": [],
"links": {
"first": "https://www.profixio.com/api/seasons/711/matches?page=1",
"last": "https://www.profixio.com/api/seasons/711/matches?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": null,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/seasons/711/matches?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/seasons/711/matches",
"per_page": 15,
"to": null,
"total": 0
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Deleted matches in a season
requires authentication
Get a list of matches in a season that has been deleted
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/seasons/711/deletedMatches?limit=15&page=1&since=2022-10-24+08%3A00%3A00" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/seasons/711/deletedMatches';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
'since' => '2022-10-24 08:00:00',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/seasons/711/deletedMatches"
);
const params = {
"limit": "15",
"page": "1",
"since": "2022-10-24 08:00:00",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 105
{
"data": [
{
"matchId": 32060432,
"tournamentId": 39751,
"deletedAt": "2025-01-27 12:00:04"
}
],
"links": {
"first": "https://www.profixio.com/api/seasons/711/deletedMatches?page=1",
"last": "https://www.profixio.com/api/seasons/711/deletedMatches?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/seasons/711/deletedMatches?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/seasons/711/deletedMatches",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Tables
Match tables for a tournament
requires authentication
Get the match tables for a tournament. The tables are grouped by match category, and by match groups.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/6/tables?matchCategory=3247289" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/6/tables';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'matchCategory' => '3247289',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/6/tables"
);
const params = {
"matchCategory": "3247289",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 104
[]
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Matches in a tournament
requires authentication
Get the matches for a tournament/league. The matches are grouped by match category, and by match groups.
Includes the matchgroups and matchcategories.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/matches?updated=2022-10-24+08%3A00%3A00&fromDate=2022-10-01&toDate=2022-10-05+14%3A40%3A00&include_referee_ids=1&matchCategory=3247289&limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/matches';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'updated' => '2022-10-24 08:00:00',
'fromDate' => '2022-10-01',
'toDate' => '2022-10-05 14:40:00',
'include_referee_ids' => '1',
'matchCategory' => '3247289',
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/matches"
);
const params = {
"updated": "2022-10-24 08:00:00",
"fromDate": "2022-10-01",
"toDate": "2022-10-05 14:40:00",
"include_referee_ids": "1",
"matchCategory": "3247289",
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 97
{
"data": [],
"links": {
"first": "https://www.profixio.com/api/tournaments/10/matches?page=1",
"last": "https://www.profixio.com/api/tournaments/10/matches?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": null,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/tournaments/10/matches?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/tournaments/10/matches",
"per_page": 15,
"to": null,
"total": 0
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Match by id
requires authentication
Get a specific match for a tournament/league.
Includes the matchgroups and matchcategories.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/matches/30843160" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/matches/30843160';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/matches/30843160"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
{
"data": {
"id": 30843160,
"txid": 44,
"number": "44",
"tournamentId": 10,
"name": "",
"gameRound": 1,
"date": "2019-11-25",
"time": "09:30:00",
"homeTeam": {
"teamRegistrationId": 721490,
"globalTeamId": null,
"name": "Croatia",
"goals": null,
"isWinner": false,
"seeding": null
},
"awayTeam": {
"teamRegistrationId": 721480,
"globalTeamId": null,
"name": "Czech Republic",
"goals": null,
"isWinner": false,
"seeding": null
},
"hasWinner": false,
"winnerTeam": null,
"field": {
"id": 1227139,
"name": "Bane1",
"arena": {
"id": 205514,
"arenaName": "Standard"
}
},
"isHidden": false,
"periodInfo": {
"periodLength": 1,
"slotLength": 15,
"numberOfPeriods": 1,
"pauseLength": 0,
"extraPeriodLength": null
},
"isGroupPlay": true,
"isLeaguePlay": false,
"isPlayoff": false,
"playoffLevel": null,
"includedInTableCalculation": true,
"matchCategory": {
"id": 1102493,
"name": "Men",
"categoryCode": "M"
},
"matchGroup": {
"id": 3247289,
"displayName": "B",
"name": "2"
},
"matchLeague": null,
"settResultsFormatted": null,
"sets": [],
"matchUrl": "http://www.profixio.com/app/uefaeurosintef/match/30843160",
"matchDataUpdated": "2025-01-05T23:03:42.936035Z",
"resultsUpdated": null,
"fieldUpdated": null,
"teamsUpdated": null,
"lineupAvailableFrom": "2019-11-25T07:30:00Z",
"datetimeStart": "2019-11-25T08:30:00Z",
"spectators": null,
"organizerGlobalClubId": null
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Update game url
requires authentication
Update the game live url a match, which will be shown as a link in the match cards.
Example request:
curl --request POST \
"https://www.profixio.com/app/api/tournaments/10/matches/30843160/game_url" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json" \
--data "{
\"ext_live_url\": \"https:\\/\\/www.youtube.com\\/watch?v=123456\"
}"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/matches/30843160/game_url';
$response = $client->post(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'ext_live_url' => 'https://www.youtube.com/watch?v=123456',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/matches/30843160/game_url"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"ext_live_url": "https:\/\/www.youtube.com\/watch?v=123456"
};
fetch(url, {
method: "POST",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
Example response (204):
Empty response
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Update match
requires authentication
Update the score for a match.
Example request:
curl --request PUT \
"https://www.profixio.com/app/api/tournaments/10/matches/30843160" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json" \
--data "{
\"result\": {
\"home\": 2,
\"away\": 1
},
\"sets\": [
{
\"home\": 21,
\"away\": 19
}
]
}"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/matches/30843160';
$response = $client->put(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'result' => [
'home' => 2,
'away' => 1,
],
'sets' => [
[
'home' => 21,
'away' => 19,
],
],
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/matches/30843160"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"result": {
"home": 2,
"away": 1
},
"sets": [
{
"home": 21,
"away": 19
}
]
};
fetch(url, {
method: "PUT",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
Example response (204):
Empty response
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Organisation
Organisation by id
requires authentication
Basic information about the current organisation.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/BC.NO.FB" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/BC.NO.FB';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/BC.NO.FB"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 117
{
"data": {
"id": "BC.NO.FB",
"name": "Bergen Challenger",
"email": "",
"country": "no",
"sports": [
{
"sportId": "FB_BC"
}
]
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Categories in a organisation
requires authentication
List of categories for an organisation.
The categories are kept between seasons.
Each league tournament must belong to a Category.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/categories?sportId=VB&limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/categories';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'sportId' => 'VB',
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/categories"
);
const params = {
"sportId": "VB",
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 116
{
"data": [
{
"id": 13,
"description": "Elitserien",
"level": 2,
"sport": "VB",
"belongsToDistrict": false,
"adminDistrictId": null
}
],
"links": {
"first": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/categories?page=1",
"last": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/categories?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/categories?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/categories",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
GlobalClubs in a organisation
requires authentication
A GlobalClub may participate in many tournaments.
See the globalClubId field on TeamRegistrations and Clubs in a tournament.
For swedish organisations, the legacyId maps to the club id in IdrottOnline
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/clubs?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/clubs';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/clubs"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 114
{
"data": [
{
"id": 2415,
"name": "Alingsås Volleybollklubb",
"shortName": "Alingsås VK",
"districtId": 55,
"legacyiD": "10461",
"updatedAt": "2025-05-20 18:01:21"
}
],
"links": {
"first": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/clubs?page=1",
"last": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/clubs?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/clubs?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/clubs",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
All players in a organisation
requires authentication
If you want to filter by club, you can use the club_id parameter. For large organisations, this can be useful to reduce the number of players returned.
Also shows current ranking points for Swedish Bordtennis
This endpoint is not paginated.
Required scope: read_all_players_in_organisation
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SBTF.SE.BT/allPlayers" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json" \
--data "{
\"club_id\": 2919
}"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SBTF.SE.BT/allPlayers';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'club_id' => 2919.0,
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SBTF.SE.BT/allPlayers"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"club_id": 2919
};
fetch(url, {
method: "GET",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 109
{
"data": [
{
"id": 80568,
"firstName": "Cristobal",
"lastName": "Smith",
"birthYear": "2024",
"birthDate": "2024-01-13",
"playerClubIdsLicensed": "2919",
"playerClubId": 2919,
"gender": "M",
"rankingPoints": 700,
"rankingPlacement": 5120,
"contractClubIds": null
},
{
"id": 75439,
"firstName": "Anais",
"lastName": "Conroy",
"birthYear": "2014",
"birthDate": "2014-11-13",
"playerClubIdsLicensed": "2919",
"playerClubId": 2919,
"gender": "M",
"rankingPoints": 1506,
"rankingPlacement": 1370,
"contractClubIds": null
}
]
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Districts
Get all districts for an organisation
requires authentication
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SHF.SE.HB/districts?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SHF.SE.HB/districts';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SHF.SE.HB/districts"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 113
{
"data": [
{
"id": 802,
"name": "Handbollförbundet Öst",
"shortname": "HF Öst",
"external_id": 268,
"postal_code": "11860",
"place": "STOCKHOLM",
"adress": "Skansbrogatan 7, Idrottens Hus",
"adress2": "",
"organisationnumber": "802001-3499"
}
],
"links": {
"first": "https://www.profixio.com/api/organisations/SHF.SE.HB/districts?page=1",
"last": "https://www.profixio.com/api/organisations/SHF.SE.HB/districts?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/organisations/SHF.SE.HB/districts?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/organisations/SHF.SE.HB/districts",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Get a district
requires authentication
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SHF.SE.HB/districts/802" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SHF.SE.HB/districts/802';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SHF.SE.HB/districts/802"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 112
{
"data": {
"id": 802,
"name": "Handbollförbundet Öst",
"shortname": "HF Öst",
"external_id": 268,
"postal_code": "11860",
"place": "STOCKHOLM",
"adress": "Skansbrogatan 7, Idrottens Hus",
"adress2": "",
"organisationnumber": "802001-3499"
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Invoices
Lists club invoices for an organisation
requires authentication
requires scope: update_invoices_fx_organisation
The API key can be configured at two levels:
- Organisation level: Returns invoices issued by the organisation (forbund)
- District level: Returns only invoices issued by the specific district
The same API endpoint and scope is used for both access levels. The filtering is automatically applied based on the API key configuration.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SBF.SE.BR/invoices?createdFromDate=2022-10-01&isExported=1&fromNumber=1&limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SBF.SE.BR/invoices';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'createdFromDate' => '2022-10-01',
'isExported' => '1',
'fromNumber' => '1',
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SBF.SE.BR/invoices"
);
const params = {
"createdFromDate": "2022-10-01",
"isExported": "1",
"fromNumber": "1",
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
{
"data": [
{
"invoice": {
"number": 4,
"date": "1992-08-12",
"due_date": "2022-11-21",
"paid_date": null,
"sent_at": null,
"paid_amount": 0,
"exported": false,
"sum": 0,
"credited_amount": 0,
"remaining_amount": 0,
"is_paid": true,
"eksport_status_code": null,
"export_status_message": null
},
"head": {
"topptekst": "dolores",
"forbund_adresse": "rem",
"forbund_postnr": "nulla",
"forbund_poststed": "sed",
"forbund_telefon": "1-409-404-8598",
"forbund_telefaks": "",
"forbund_epost": "[email protected]",
"forbund_hjemmeside": "perferendis",
"forbund_iban": "",
"forbund_swift": "",
"forbund_organisasjonsnummer": "63171552",
"forbund_konto": "",
"id": 34597,
"klubbid": 30583,
"fakturanr": 4,
"eksternid": 30583,
"navn": "Willmsfurt",
"kontaktnavn": "",
"adresse": "delectus",
"postnr": "ducimus",
"poststed": "nulla",
"opprettetdato": "1992-08-12",
"forfalldato": "2022-11-21"
},
"lines": []
},
{
"invoice": {
"number": 766,
"date": "1999-07-15",
"due_date": "2022-03-17",
"paid_date": null,
"sent_at": null,
"paid_amount": 0,
"exported": false,
"sum": 0,
"credited_amount": 0,
"remaining_amount": 0,
"is_paid": true,
"eksport_status_code": null,
"export_status_message": null
},
"head": {
"topptekst": "dolorum",
"forbund_adresse": "cupiditate",
"forbund_postnr": "non",
"forbund_poststed": "perspiciatis",
"forbund_telefon": "+1.283.466.6935",
"forbund_telefaks": "",
"forbund_epost": "[email protected]",
"forbund_hjemmeside": "est",
"forbund_iban": "",
"forbund_swift": "",
"forbund_organisasjonsnummer": "78552765",
"forbund_konto": "",
"id": 34598,
"klubbid": 30584,
"fakturanr": 766,
"eksternid": 30584,
"navn": "Laishaview",
"kontaktnavn": "",
"adresse": "nulla",
"postnr": "itaque",
"poststed": "illo",
"opprettetdato": "1999-07-15",
"forfalldato": "2022-03-17"
},
"lines": []
}
]
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Show a specific invoice
requires authentication
requires scope: update_invoices_fx_organisation
The API key access level (organisation or district) determines which invoices can be accessed.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SBF.SE.BR/invoices/123456" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SBF.SE.BR/invoices/123456';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SBF.SE.BR/invoices/123456"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
{
"data": {
"invoice": {
"number": 933724,
"date": "2007-08-14",
"due_date": "2015-01-19",
"paid_date": null,
"sent_at": null,
"paid_amount": 0,
"exported": false,
"sum": 0,
"credited_amount": 0,
"remaining_amount": 0,
"is_paid": true,
"eksport_status_code": null,
"export_status_message": null
},
"head": {
"topptekst": "mollitia",
"forbund_adresse": "accusamus",
"forbund_postnr": "doloribus",
"forbund_poststed": "fugit",
"forbund_telefon": "+1 (216) 979-0089",
"forbund_telefaks": "",
"forbund_epost": "[email protected]",
"forbund_hjemmeside": "qui",
"forbund_iban": "",
"forbund_swift": "",
"forbund_organisasjonsnummer": "5110483",
"forbund_konto": "",
"id": 34599,
"klubbid": 30585,
"fakturanr": 933724,
"eksternid": 30585,
"navn": "Raynormouth",
"kontaktnavn": "",
"adresse": "ex",
"postnr": "aut",
"poststed": "qui",
"opprettetdato": "2007-08-14",
"forfalldato": "2015-01-19"
},
"lines": []
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Bulk update
requires authentication
Update the exported status, and paid status of invoices
- After importing invoices, set export status to 200 if successful, 400 if failed.
- After receiving payment, set paid date and amount.
requires scope: update_invoices_fx_organisation
Only invoices accessible to the API key (based on organisation or district level) can be updated.
Example request:
curl --request POST \
"https://www.profixio.com/app/api/organisations/BC.NO.FB/invoices/bulkUpdate" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json" \
--data "{
\"invoices\": [
{
\"number\": 107,
\"export_status_code\": 200,
\"export_status_message\": \"Invoice 107 created in TotalInvoice\",
\"paid_date\": \"2021-01-01\",
\"paid_amount\": 100,
\"credited_amount\": 100
}
]
}"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/BC.NO.FB/invoices/bulkUpdate';
$response = $client->post(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'json' => [
'invoices' => [
[
'number' => 107,
'export_status_code' => 200,
'export_status_message' => 'Invoice 107 created in TotalInvoice',
'paid_date' => '2021-01-01',
'paid_amount' => 100.0,
'credited_amount' => 100.0,
],
],
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/BC.NO.FB/invoices/bulkUpdate"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
let body = {
"invoices": [
{
"number": 107,
"export_status_code": 200,
"export_status_message": "Invoice 107 created in TotalInvoice",
"paid_date": "2021-01-01",
"paid_amount": 100,
"credited_amount": 100
}
]
};
fetch(url, {
method: "POST",
headers,
body: JSON.stringify(body),
}).then(response => response.json());
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Ranking
RankingPoints for a tournament
requires authentication
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/rankingpoints" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/rankingpoints';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/rankingpoints"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
[
{
"lag_id": 1080287,
"fk_klasse": 159911,
"lag_klasse": "D",
"entrypoeng": 333,
"entrypoeng_sort": 333.00000245,
"playerpoints": {
"89861": 88,
"43821": 245
}
}
]
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
RankingPoints for a team
requires authentication
Only returns data if the tournament uses ranking. Mostly used for volleyball (NVBF / SVBF)
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/rankingpoints/721480" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/rankingpoints/721480';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/rankingpoints/721480"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
{
"lag_id": 1080287,
"fk_klasse": 159911,
"lag_klasse": "D",
"entrypoeng": 333,
"entrypoeng_sort": 333.00000245,
"playerpoints": {
"89861": 88,
"43821": 245
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Season
Seasons in a organisation
requires authentication
List of seasons for an organisation
Sorting: Newest seasons first
Response fields
currentlyActive describes if this is the active season in Profixio for this organisation.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/seasons?sportId=VB&limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/seasons';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'sportId' => 'VB',
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/seasons"
);
const params = {
"sportId": "VB",
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 115
{
"data": [
{
"id": 751,
"name": "Säsongen 2024/25",
"sportId": "VB",
"currentlyActive": true,
"seasonStartDate": "2024-05-16",
"seasonEndDate": "2025-05-31",
"registrationStartDate": null,
"registrationLastDate": null
}
],
"links": {
"first": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/seasons?page=1",
"last": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/seasons?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/seasons?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/seasons",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Season by Id
requires authentication
Return information about a specific season by Id
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/seasons/architecto" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/seasons/architecto';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/seasons/architecto"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
{
"data": {
"id": 762,
"name": "adipisci",
"sportId": "FB",
"currentlyActive": true,
"seasonStartDate": "2025-05-16",
"seasonEndDate": "2025-02-17",
"registrationStartDate": "2025-02-09",
"registrationLastDate": "2025-04-17"
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
The season tree
requires authentication
Lists categories, divisions and tournaments for a season.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/seasons/711/tree?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/seasons/711/tree';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/seasons/711/tree"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 108
[
{
"id": 496,
"name": "Förbundsserier",
"level": 10,
"sport": "BB",
"belongsToDistrict": false,
"adminDistrictId": null,
"divisions": [
{
"id": 4533,
"name": "Herrar",
"belongsToDistrict": false,
"adminDistrictId": null,
"tournaments": [
{
"id": 30963,
"name": "SBL Herr",
"slug": "leagueid13505",
"webUrl": "https://www.profixio.com/app/leagueid13505",
"matchCount": 196,
"belongsToDistrict": false,
"adminDistrictId": null,
"matchCategories": [
{
"id": 1124523,
"name": "SBL Herr",
"categoryCode": "SBL Herr"
}
]
}
]
}
]
}
]
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Statistics
Tournament
Playerstatistics
requires authentication
Get player statistics in a series. The player statistics are collected from the matches with livescore, after the match has finished.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/playerStats?matchCategory=3247289" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/playerStats';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'matchCategory' => '3247289',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/playerStats"
);
const params = {
"matchCategory": "3247289",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
{
"data": [
{
"teamId": 1410369,
"globalClubId": 33364617,
"tournamentId": 40485,
"teamName": "West Mableview",
"personId": 1048546,
"firstName": "Marley",
"lastLame": "Reichel",
"assists": 1,
"totalGoals": 5,
"gameGoals": 3,
"penaltyGoals": 2,
"games": 3,
"gamesPlayed": 2
},
{
"teamId": 1410370,
"globalClubId": 7,
"tournamentId": 40492,
"teamName": "Ondrickaburgh",
"personId": 1048547,
"firstName": "Cruz",
"lastLame": "O'Reilly",
"assists": 1,
"totalGoals": 5,
"gameGoals": 3,
"penaltyGoals": 2,
"games": 3,
"gamesPlayed": 2
}
]
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Tournament
Tournaments
Tournaments in a organisation
requires authentication
List of tournament (not leagues) for an organisation
Only lists tournament newer than 1 year ago.
Sorting: By start date, newest first
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/tournaments?sportId=VB&limit=15&page=1&open_registration=16" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/tournaments';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'sportId' => 'VB',
'limit' => '15',
'page' => '1',
'open_registration' => '16',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/organisations/SVBF.SE.SVB/tournaments"
);
const params = {
"sportId": "VB",
"limit": "15",
"page": "1",
"open_registration": "16",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 110
{
"data": [],
"links": {
"first": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/tournaments?page=1",
"last": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/tournaments?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": null,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/tournaments?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/organisations/SVBF.SE.SVB/tournaments",
"per_page": 15,
"to": null,
"total": 0
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Leagues
Tournaments in a season
requires authentication
List of tournaments/leagues for a season.
Can be filtered by category and/or sport.
TIP: Also see 'The season tree' endpoint to get all leagues, sorted under divisions and categories.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/seasons/711/tournaments?limit=15&page=1&categoryId=496&sportId=BB" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/seasons/711/tournaments';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
'categoryId' => '496',
'sportId' => 'BB',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/seasons/711/tournaments"
);
const params = {
"limit": "15",
"page": "1",
"categoryId": "496",
"sportId": "BB",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 107
{
"data": [
{
"id": 30963,
"name": "SBL Herr",
"categoryId": 496,
"categoryName": "Förbundsserier",
"divisionId": 4533,
"divisionName": "Herrar",
"seasonId": 711,
"matchCount": 196,
"registrationTeamCount": 11,
"matchCategories": [
{
"id": 1124523,
"name": "SBL Herr",
"categoryCode": "SBL Herr"
}
]
}
],
"links": {
"first": "https://www.profixio.com/api/seasons/711/tournaments?page=1",
"last": "https://www.profixio.com/api/seasons/711/tournaments?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/seasons/711/tournaments?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/seasons/711/tournaments",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Tournament by id
requires authentication
Info about a tournament
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/architecto" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/architecto';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/architecto"
);
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
{
"data": {
"id": 40493,
"name": "aut adipisci quidem2025",
"slug": "autadipisciquidem2025",
"isTournament": true,
"webUrl": "http://www.profixio.com/app/autadipisciquidem2025",
"organisationId": "NFF.NO.FB",
"countryId": "NO",
"startDate": "2025-06-27",
"endDate": "2025-06-30",
"language": "",
"isDeactivated": false,
"updatedAt": "2025-05-27T12:32:12.000000Z",
"isCancelled": false,
"usesClub": true,
"clubName": "North Roderick",
"tournamentIsFinished": false,
"tournamentHasStarted": false,
"sport": {
"id": "FB",
"name": "Fotball",
"sportsnamePublic": "Fotball"
},
"contactinfo": {
"email": "[email protected]",
"address": "",
"postalCode": "06227",
"place": "Lucienne Curve",
"phone": "",
"homepage": "http://www.dare.org/iure-odit-et-et-modi-ipsum-nostrum-omnis"
},
"teamRegistrationConfig": {
"registrationIsOpen": true,
"registrationFromDate": null,
"registrationLastDate": "2025-07-03T12:32:12.000000Z",
"publicDeadline": "2025-07-03T12:32:12.000000Z",
"hideRegistrationsPublic": false,
"registrationsCount": 0
},
"servicesConfig": {
"registrationIsOpen": false,
"hasServices": false,
"deadline": "2025-07-06T22:00:00.000000Z"
},
"playerRegistrationConfig": {
"registrationIsOpen": true,
"deadline": "2025-07-05"
},
"refereeConfig": {
"registrationIsOpen": false,
"registrationStartDate": "2025-06-27",
"refereeRegistrationDeadline": null
},
"matchScheduleConfig": {
"hideMatches": false,
"status": "UNPUBLISHED",
"categoriesCount": 0,
"matchesCount": 0
}
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
TeamRegistrations in a tournament
requires authentication
Get teams registered in a tournament. The team registrations are done before matches are published.
The id field here maps to teamRegistrationId on the home and awayteams in a Match
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/teams?players=&seeding=&limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/teams';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'players' => '0',
'seeding' => '0',
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/teams"
);
const params = {
"players": "0",
"seeding": "0",
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 103
{
"data": [
{
"id": 721480,
"name": "Czech Republic",
"clubId": 175423,
"globalClubId": null,
"registrationClass": 119453,
"registrationClassCode": "M",
"matchCategories": [
{
"id": 1102493
}
],
"registrationLevel": null,
"jersey": "",
"onWaitingList": false,
"statusCode": 0,
"registeredAt": "2017-07-10T09:48:26.000000Z",
"deletedAt": null,
"updatedAt": "2017-07-10 09:48:26",
"isDeactivatedInSchedule": false,
"countryId": null,
"registrationFeeStatus": {
"isPaid": true,
"baseFee": 0,
"discount": 0,
"amount": 0,
"paid": 0
},
"serviceFeeStatus": {
"isPaid": true,
"amount": 0,
"ordersCount": 0,
"paid": 0
},
"registrationComment": "",
"teamUrl": "https://www.profixio.com/app/uefaeurosintef/teams/721480"
}
],
"links": {
"first": "https://www.profixio.com/api/tournaments/10/teams?page=1",
"last": "https://www.profixio.com/api/tournaments/10/teams?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/tournaments/10/teams?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/tournaments/10/teams",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
GlobalTeams in a tournament
requires authentication
Get teams for a tournament for Leagues which do not use TeamRegistration.
The id field here maps to globalTeamId on the home and awayteams in a Match
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/globalTeams?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/globalTeams';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/globalTeams"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 102
{
"data": [
{
"id": 14655,
"name": "Broberg/Söderhamn IF",
"globalClubId": 23175
}
],
"links": {
"first": "https://www.profixio.com/api/tournaments/10/globalTeams?page=1",
"last": "https://www.profixio.com/api/tournaments/10/globalTeams?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/tournaments/10/globalTeams?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/tournaments/10/globalTeams",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Clubs in a tournament
requires authentication
Get clubs for a tournament. Only returns clubs that has teamRegistrations
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/clubs?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/clubs';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/clubs"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 101
{
"data": [
{
"id": 175423,
"name": "NoName",
"globalClubId": null,
"tournamentId": 10,
"countryId": null
}
],
"links": {
"first": "https://www.profixio.com/api/tournaments/10/clubs?page=1",
"last": "https://www.profixio.com/api/tournaments/10/clubs?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/tournaments/10/clubs?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/tournaments/10/clubs",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
MatchCategories in a tournament
requires authentication
Get MatchCategories for a tournament.
A matchcategory can have:
- MatchGroups (Pulje)
- MatchLeagues (SluttspillSerie / MellomspillSerie)
- Playoff (Sluttspill)
In Playoffs the teams are automatically placed based on the results from the group and league matches.
Example:
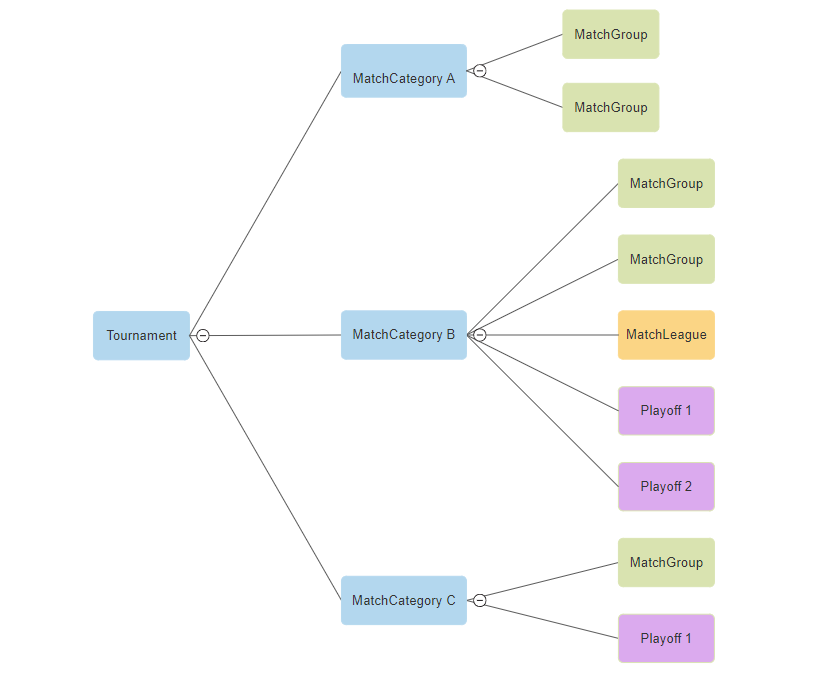
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/matchCategories?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/matchCategories';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/matchCategories"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 100
{
"data": [
{
"id": 1102493,
"name": "Men",
"categoryCode": "M",
"numberOfSets": 0,
"sortOrder": 0,
"matchlength": 15,
"matchtableUpdatedAt": "2019-09-03T15:11:09.000000Z",
"matchTableIsHidden": false,
"numberOfPeriods": 1,
"isHidden": false,
"hideResults": false,
"gender": "M",
"minutesPerPeriod": 1,
"poolPlay": 0,
"matchGroups": [
{
"id": 3247288,
"matchCategoryId": 1102493,
"displayName": "A",
"name": "1",
"sortOrder": 0,
"matchtableUpdatedAt": "0000-12-31T23:17:00.000000Z"
},
{
"id": 3247289,
"matchCategoryId": 1102493,
"displayName": "B",
"name": "2",
"sortOrder": 0,
"matchtableUpdatedAt": "0000-12-31T23:17:00.000000Z"
},
{
"id": 3247290,
"matchCategoryId": 1102493,
"displayName": "C",
"name": "3",
"sortOrder": 0,
"matchtableUpdatedAt": "0000-12-31T23:17:00.000000Z"
},
{
"id": 3247291,
"matchCategoryId": 1102493,
"displayName": "D",
"name": "4",
"sortOrder": 0,
"matchtableUpdatedAt": "0000-12-31T23:17:00.000000Z"
}
],
"matchLeagues": [
{
"id": 3313,
"name": "L A",
"sortOrder": 100
},
{
"id": 3311,
"name": "L B",
"sortOrder": 100
},
{
"id": 3312,
"name": "L C",
"sortOrder": 100
}
],
"playoffTrees": [
{
"id": 55087,
"published": true,
"name": "",
"number": 1
}
]
}
],
"links": {
"first": "https://www.profixio.com/api/tournaments/10/matchCategories?page=1",
"last": "https://www.profixio.com/api/tournaments/10/matchCategories?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/tournaments/10/matchCategories?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/tournaments/10/matchCategories",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
MatchGroups in a tournament
requires authentication
Get the MatchGroups in a tournament. Each MatchGroup belongs to a MatchCategory
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/10/matchGroups?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/10/matchGroups';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/10/matchGroups"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 99
{
"data": [
{
"id": 3247288,
"matchCategoryId": 1102493,
"displayName": "A",
"name": "1",
"sortOrder": 0,
"matchtableUpdatedAt": "0000-12-31T23:17:00.000000Z"
}
],
"links": {
"first": "https://www.profixio.com/api/tournaments/10/matchGroups?page=1",
"last": "https://www.profixio.com/api/tournaments/10/matchGroups?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/tournaments/10/matchGroups?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/tournaments/10/matchGroups",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.
Arenas
Arenas for a tournament
requires authentication
Get the arenas for a tournament. The arenas are grouped by match category, and by match groups.
Example request:
curl --request GET \
--get "https://www.profixio.com/app/api/tournaments/6/arenas?limit=15&page=1" \
--header "X-Api-Secret: {YOUR_AUTH_KEY}" \
--header "Content-Type: application/json" \
--header "Accept: application/json"
$client = new \GuzzleHttp\Client();
$url = 'https://www.profixio.com/app/api/tournaments/6/arenas';
$response = $client->get(
$url,
[
'headers' => [
'X-Api-Secret' => '{YOUR_AUTH_KEY}',
'Content-Type' => 'application/json',
'Accept' => 'application/json',
],
'query' => [
'limit' => '15',
'page' => '1',
],
]
);
$body = $response->getBody();
print_r(json_decode((string) $body));
const url = new URL(
"https://www.profixio.com/app/api/tournaments/6/arenas"
);
const params = {
"limit": "15",
"page": "1",
};
Object.keys(params)
.forEach(key => url.searchParams.append(key, params[key]));
const headers = {
"X-Api-Secret": "{YOUR_AUTH_KEY}",
"Content-Type": "application/json",
"Accept": "application/json",
};
fetch(url, {
method: "GET",
headers,
}).then(response => response.json());
Example response (200):
Show headers
cache-control: no-cache, private
content-type: application/json
x-ratelimit-limit: 120
x-ratelimit-remaining: 98
{
"data": [
{
"id": 198159,
"name": "Älta IP",
"fields": [
{
"id": 1193360,
"name": "Plan 2"
},
{
"id": 1193361,
"name": "Plan 3"
}
]
}
],
"links": {
"first": "https://www.profixio.com/api/tournaments/6/arenas?page=1",
"last": "https://www.profixio.com/api/tournaments/6/arenas?page=1",
"prev": null,
"next": null
},
"meta": {
"current_page": 1,
"from": 1,
"last_page": 1,
"links": [
{
"url": null,
"label": "Prev",
"active": false
},
{
"url": "https://www.profixio.com/api/tournaments/6/arenas?page=1",
"label": "1",
"active": true
},
{
"url": null,
"label": "Next",
"active": false
}
],
"path": "https://www.profixio.com/api/tournaments/6/arenas",
"per_page": 15,
"to": 1,
"total": 1
}
}
Received response:
Request failed with error:
Tip: Check that you're properly connected to the network.
If you're a maintainer of ths API, verify that your API is running and you've enabled CORS.
You can check the Dev Tools console for debugging information.